Unityではテキストはstringしか受け付けないとか、座標はfloatで返るとかいろいろとデータ型縛りがあり、取得した値を別のデータ型に置き換える場面が多くでてきます。
そんなわけで各種データ型変更方法まとめです。
前置き
動作確認として、データ型の確認を行うGetTypeを使用します。
stringに変換
ToString(なんでも→string)
stringに変換
変数.ToString()
変数.ToString()
//Unity C#
void Start()
{
int a = 1;
//int→string
string b = a.ToString();
Debug.Log(b);
Debug.Log(b.GetType());
}
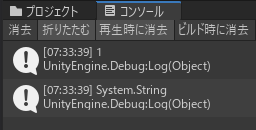
他の形式のデータをstring形式に変換するには、ToStringメソッドを使用します。intやfloatはもちろん、bool値も文字列に変換できます(True、Falseという文字列になる)。
ToStringした数値の桁指定
//Unity C#
int a = 1;
string b = a.ToString("0000");
ToStringで数値を変換する時に、最低桁数を0の数で指定することができます。
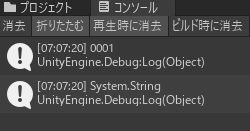
0000で指定すればこうなります。10000以上は普通に桁数が増えます。
//Unity C#
int a = 1;
string b = a.ToString(".0000");
同様に0.0000とか、.0000で指定してやれば、
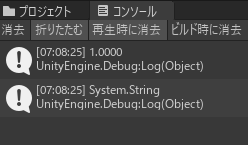
小数点以下の最低桁数を指定することもできます。
Convert.ToString(なんでも→string)
stringに変換
Convert.ToString(変数)
Convert.ToString(変数)
//Unity C#
using System;
void Start()
{
bool a = true;
//bool→string
string b = Convert.ToString(a);
Debug.Log(b);
Debug.Log(b.GetType());
}
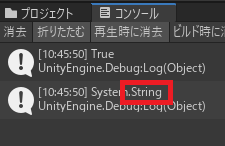
ToStringと同じような感じで使えますが、名前空間でusing System;を宣言する必要があります。
以下それぞれ書いてますが、Convert Toの後にGetTypeで取得できるデータ型(図赤枠部分)を付ければ、int、float、double、boolどれでも変換できます(Convert ToInt32()など)
以下それぞれ書いてますが、Convert Toの後にGetTypeで取得できるデータ型(図赤枠部分)を付ければ、int、float、double、boolどれでも変換できます(Convert ToInt32()など)
intに変換
int.Parse(string→int)
string→intに変換
int.Parse(変数)
int.Parse(変数)
//Unity C#
void Start()
{
string a = "1";
//string→int
int b = int.Parse(a);
Debug.Log(b);
Debug.Log(b.GetType());
}
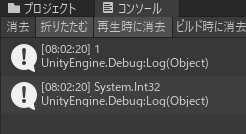
int.Parseを使うと、string形式の整数をint形式に変換することができます。
floatなどには使用できません。
floatなどには使用できません。
int(float/double→int)
intに変換(小数切り捨て)
(int)変数
(int)変数
//Unity C#
void Start()
{
float a = 1.99f;
//float→int(小数切り捨て)
int b = (int)a;
Debug.Log(b);
Debug.Log(b.GetType());
}
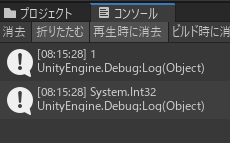
頭に(int)を付けることで、float、double値を整数にすることができます。小数は切り捨てられます。
stringなどは変換できません。
stringなどは変換できません。
Mathf(float→int)
float→intに変換(小数切り上げ)
Mathf.CeilToInt(変数)
Mathf.CeilToInt(変数)
float→intに変換(小数切り捨て)
Mathf.FloorToInt(変数)
float→intに変換(偶数丸め)
Mathf.RoundToInt(変数)
//Unity C#
float a = 1.5f;
//float→int(小数切り上げ)
int b = Mathf.CeilToInt(a);
Debug.Log(b);
Debug.Log(b.GetType());
//float→int(小数切り捨て)
int c = Mathf.FloorToInt(a);
Debug.Log(c);
Debug.Log(c.GetType());
//float→int(偶数丸め)
int d = Mathf.RoundToInt(a);
Debug.Log(d);
Debug.Log(d.GetType());
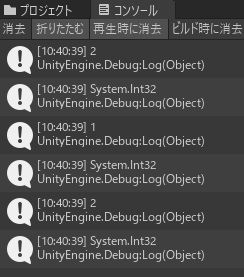
Mathf.Ceil、Floor、RoundにToIntをつけることで、小数を切り上げたり切り下げたりしたint値を戻すことができます。
Mathfの挙動、偶数丸めなどについて詳しくはこちら。
Convert.ToInt32(なんでも→int)
intに変換
Convert.ToInt32(変数)
Convert.ToInt32(変数)
//Unity C#
using System;
void Start()
{
bool a = true;
//bool→int(Trueは1、Falseは0)
int b = Convert.ToInt32(a);
Debug.Log(b);
Debug.Log(b.GetType());
}
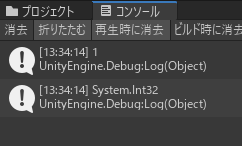
Convert.ToInt32を使うには、名前空間でusing System;を記述する必要があります。
boolを0か1に変換できる他、floatかdoubleなら偶数丸め、stringならただの整数のみ変換できます(0.00とかはダメ)
boolを0か1に変換できる他、floatかdoubleなら偶数丸め、stringならただの整数のみ変換できます(0.00とかはダメ)
floatに変換
float.Parse(string→float)
string→floatに変換
float.Parse(変数)
float.Parse(変数)
//Unity C#
void Start()
{
string a = "1";
//string→float
float b = float.Parse(a);
Debug.Log(b);
Debug.Log(b.GetType());
}
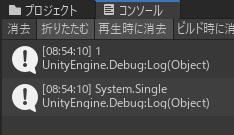
同様に、float.Parseを使うとstring形式の整数をfloat形式に変換することができます。元の文字列にfは不要です。
int→floatなどはできません。
int→floatなどはできません。
float(int/double→float)
floatに変換
(float)変数
(float)変数
//Unity C#
void Start()
{
double a = 1.123456789;
//double→float
float b = (float)a;
Debug.Log(b);
Debug.Log(b.GetType());
}
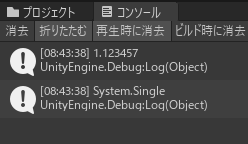
int同様、頭に(float)を付けることで、int、double値をfloat値にすることができます。stringなどは変換できません。
Convert.ToSingle(なんでも→float)
floatに変換
Convert.ToSingle(変数)
Convert.ToSingle(変数)
//Unity C#
using System;
void Start()
{
bool a = true;
//bool→float
float b = Convert.ToSingle(a);
Debug.Log(b);
Debug.Log(b.GetType());
}
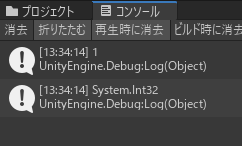
名前空間でusing System;を宣言する必要があります。
int、double、boolをfloatに変換できます。stringの場合、
int、double、boolをfloatに変換できます。stringの場合、
"1"
、"1.1"
などはOKですが、"1f"
や数字以外の文字列はエラーになります。doubleに変換
double(int/float→double)
doubleに変換
(double)変数
(double)変数
//Unity C#
void Start()
{
float a = 1.123456789f;
//float→double
double b = (double)a;
Debug.Log(b);
Debug.Log(b.GetType());
}
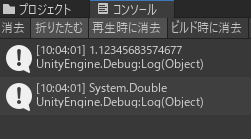
(double)を付けることで、int、float値をdouble値にすることができます。
stringなどは変換できません。
stringなどは変換できません。
Convert.ToDouble(なんでも→double)
doubleに変換
Convert.ToDouble(変数)
Convert.ToDouble(変数)
//Unity C#
using System;
void Start()
{
string a = "1.2345";
//string→double
double b = Convert.ToDouble(a);
Debug.Log(b);
Debug.Log(b.GetType());
}
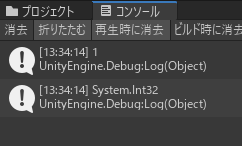
名前空間でusing System;を宣言する必要があります。
int、float、boolをdoubleに変換できます。stringの場合、数字以外の文字列はエラーになります。
int、float、boolをdoubleに変換できます。stringの場合、数字以外の文字列はエラーになります。
boolに変換
Convert.ToBoolean(なんでも→bool)
boolに変換
Convert.ToBoolean(変数)
Convert.ToBoolean(変数)
//Unity C#
using System;
void Start()
{
int a = 1;
//int→bool(0=False、それ以外=True)
bool b = Convert.ToBoolean(a);
Debug.Log(b);
Debug.Log(b.GetType());
}
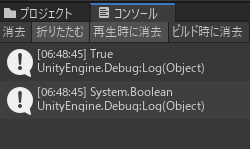
Convert.ToBooleanを噛ませることで、int、float、doubleをbool値にすることができます。Convertクラスを使う場合、名前空間でusing System;を記述する必要があります。
試した感じ0以外は全部Trueになるようです。1も300も-0.5fもTrueです。
試した感じ0以外は全部Trueになるようです。1も300も-0.5fもTrueです。
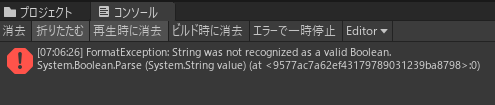
stringの場合、”true””True””tRue”などはTrueに、”false””False””FALSE”などはFalseになります。他の文字列および”1″、”0″はエラーになります。
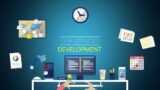
やりたいことから逆引きするUNITYの使い方まとめ
Unityをやりたいことから学習していけるよう、機能・用途別にまとめたページです。C#の命令別の逆引きは現時点で作っていません。2019の時期に書き始めているので、それより前のバージョンについては言及しません。
コメント